The new project of Arduino with DHT11 temperature & humidity sensor module. This sensor is pre-measured and don’t need extra component so you can start measuring temperature and humidity right away.
How DHT11 Measures Temperature and Humidity
Inside DHT11 there is humidity sensor along with Thermistor.
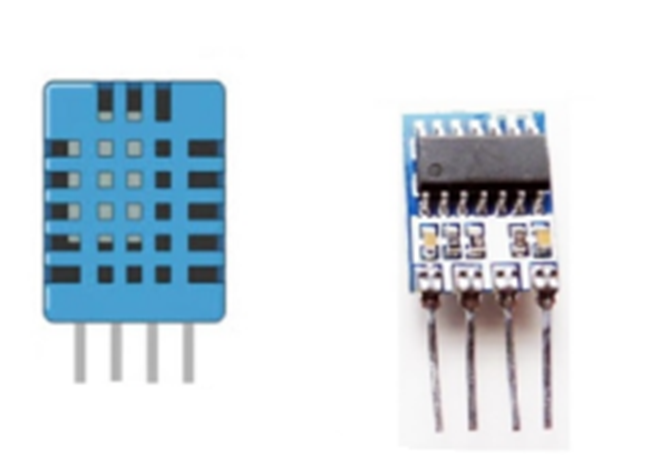
Humidity sensing component has two electrodes with moisture holding substrate sandwiched between them.
The ions are released by the substrate as water vapor is absorbed by it, which increases the conductivity between the electrodes.
The change in resistance between two electrodes is proportional to the relative humidity. Higher humidity decreases the resistance between these electrodes, while lower humidity increases the resistance between the electrodes.
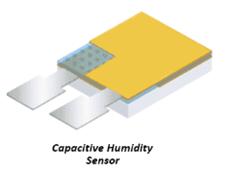
DHt11 also contains a NTC/Thermistor to measure temperature. A thermistor is a thermal resistor whose resistance changes with temperature. The term “NTC” means “Negative Temperature Coefficient”, which means that temperature increases with decrease in resistance.
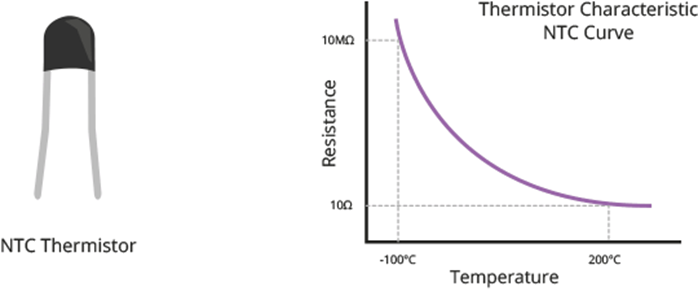
On the other side, there is a small PCB with an 8-bit SOIC-14 packaged IC. This IC measures and processes the analog signal with stored calibration coefficients, does analog to digital conversion and spits out a digital signal with the temperature and humidity.
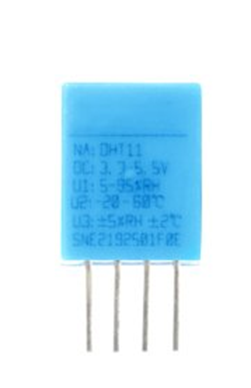
DHT11 Sensor
DHT11 can measure temperature from 0°C to 50°C with ±2.0°C accuracy, and humidity from 20 to 80% with 5% accuracy.
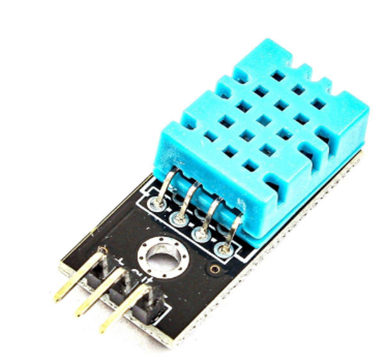
The sampling rate of the DHT11 is 1Hz, means that you can get new data from it once every second.
DHT11 Module Pinout
The DHT11 module is easy to connect. It has three pins:
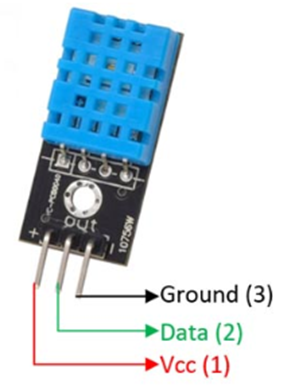
+(VCC) pin supplies power for the sensor. 5V supply is recommended, although the supply voltage ranges from 3.3V to 5.5V.
Out pin is used to communication between the Arduino and the sensor.
– (GND) should be connected to the ground of Arduino.
Wiring DHT11 Module to Arduino
Connections are very simple. The VCC pin of sensor is connected with 5V of Arduino. And GND of sensor is connected with the GND. Finally, the out pin of sensor is connected to the digital pin # 8.
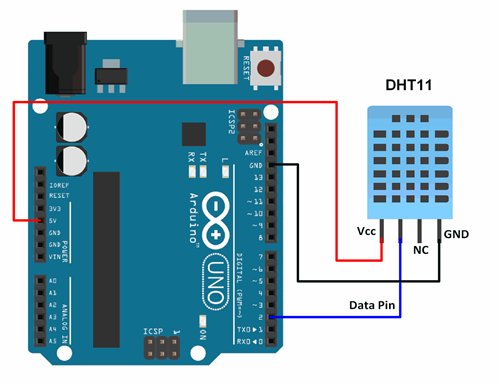
Installing DHT library
DHT11 sensors have their own single wire protocol for transferring the data. This protocol requires precise timing. Fortunately, DHT Library was written to hide away all the complexities so that we can issue simple commands to read the temperature and humidity data.
Download the library first by click on the link https://github.com/adidax/dht11.
To install it, open the Arduino IDE, go to Sketch > Include Library > Add .ZIP Library, and then select the DHTlib ZIP file that you just downloaded.
Arduino Code – Basic Example
Once you installed the library, you can copy this code into Arduino ide. This code will print the value of temperature and humidity on the Serial monitor.
#include <dht.h> // Include library
#define outPin 8 // Defines pin number to which the sensor is connected
dht DHT; // Creates a DHT object
void setup() {
Serial.begin(9600);
}
void loop() {
int readData = DHT.read11(outPin);
float t = DHT.temperature; // Read temperature
float h = DHT.humidity; // Read humidity
Serial.print("Temperature = ");
Serial.print(t);
Serial.print("°C | ");
Serial.print((t*9.0)/5.0+32.0); // Convert celsius to fahrenheit
Serial.println("°F ");
Serial.print("Humidity = ");
Serial.print(h);
Serial.println("% ");
Serial.println("");
delay(2000); // wait two seconds
}
The output on Serial monitor is:
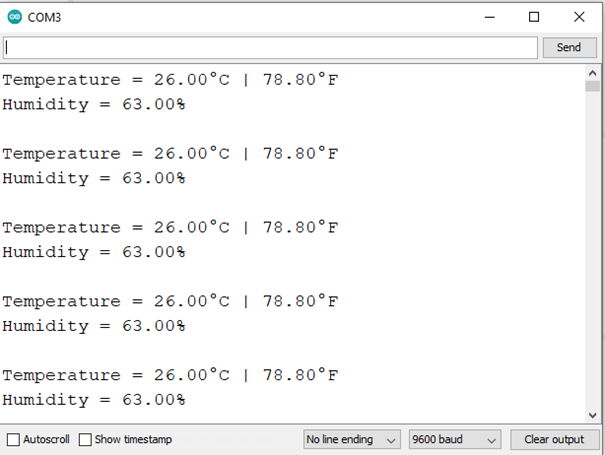
Code Explanation:
First of all we include the library of DHT11 and define Arduino pin to which sensor is connected. Then we create a DHT object to access special functions related to the library.
#include
#define outPin 8
dht DHT;
In void setup function: we will initialize Serial monitor to do communication
void setup() {
Serial.begin(9600);
}
In the void loop function; we will use the read11() function which reads the data from the sensor. It takes sensor’s Data pin number as a parameter. And store it in readData Variable.
int readData = DHT.read11(outPin);
Once the humidity and temperature values are calculated, we can use them by:
float t = DHT.temperature; // Read temperature
float h = DHT.humidity; // Read humidity
The DHT object only return value of temperature in Celsius (°C). If we want temperature in Fahrenheit (°F), than we have to use this formula:
Serial.print((t * 9.0) / 5.0 + 32.0);