LM35 is another cheap and small sensor that you can use in your projects. You don’t need any other components to work with this sensor. Some wiring and Arduino code is enough for this that we will discuss in this tutorial.
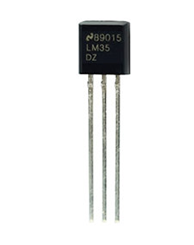
This sensor is fairly precise and cheap. It is accurate and works with different environmental conditions. LM35 sensor does not require calibration and provides accuracy of ±0.5°C at room temperature and ±1°C over a full −55°C to +155°C temperature range.
Some specification on this sensors are as follows:
Power supply | 4V to 30V |
Current draw | 60µA |
Temperature range | −55°C to +155°C |
Accuracy | ±0.5°C |
Output scale factor | 10mV/°C |
Output at 25°C | 250mV |
The disadvantage of this sensor is that it cannot measure temperature without negative biasing. For measuring negative temperature we recommend you to use another sensor (TMP36).
Working Principle of LM35 sensor
It uses a solid-state method to measure the temperature. It makes use of the fact that the voltage drop between the base and emitter (forward voltage – Vbe) of the Diode-connected transistor decreases at a known rate as the temperature increases. By amplifying this voltage change, it is possible to generate analog signal that is directly proportional to the temperature.
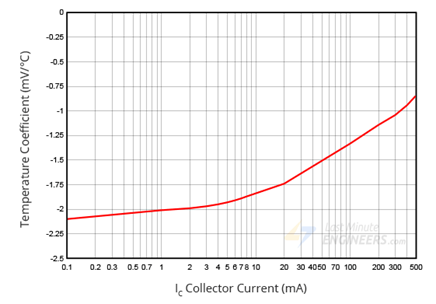
The good thing is that these calculations are done by sensor itself it shows only voltage only that is directly proportional to the temperature.
How to Measure Temperature
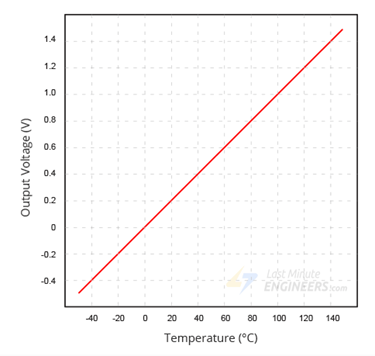
To convert voltage to temperature we use simple formula:
Temperature (°C) = Vout * 100
For example, if the voltage out is 0.5V that means that the temperature is 0.5 * 100 = 50 °C
For example you want to find temperature, touch the sensor with ice and checks temperature.
LM35 Sensor Pinout
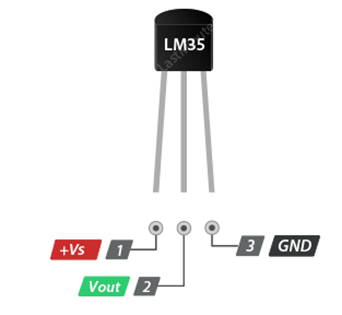
+Vs is the power supply which can be between 4V to 30V.
Vout pin produces an analog voltage that is directly proportional to the temperature. It should be connected to an Analog input.
GND is a ground pin.
Connecting the LM35 Temperature Sensor to an Arduino
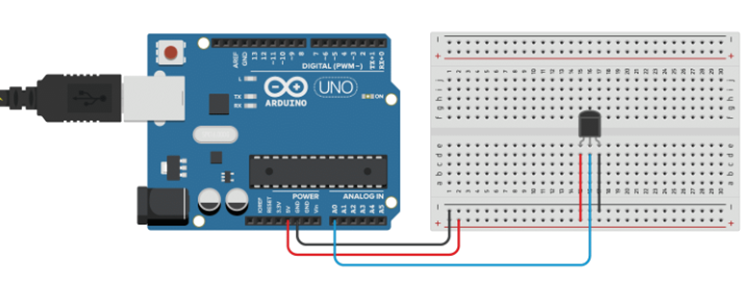
You need to connect left wire with positive 5V of the Arduino. Right wire to the negative pin and the middle pin goes to the analog input pin.
Reading the Analog Temperature Data
As we can see one of the pin of sensor is connected to the Arduino analog pin. We can read value from analog read by using function analogRead ().
Analog read function will not give the voltages between o-5 it will send data from 0-1023. We have to convert this data in between 0-5 for further processing.
We use this simple formula to convert it in volts.
Vout = (reading from ADC) * (5 / 1024)
This formula convert the values between 0-5V so we can calculate exact temperature by using this voltage.
The formula for temperature is:
Temperature (°C) = Vout * 100
Arduino Code – Simple Thermometer
// Define the analog pin, the LM35's Vout pin is connected to
#define sensorPin A0
void setup() {
// Begin serial communication at 9600 baud rate
Serial.begin(9600);
}
void loop() {
// Get the voltage reading from the LM35
int reading = analogRead(sensorPin);
// Convert that reading into voltage
float voltage = reading * (5.0 / 1024.0);
// Convert the voltage into the temperature in Celsius
float temperatureC = voltage * 100;
// Print the temperature in Celsius
Serial.print("Temperature: ");
Serial.print(temperatureC);
Serial.print("\xC2\xB0"); // shows degree symbol
Serial.print("C | ");
// Print the temperature in Fahrenheit
float temperatureF = (temperatureC * 9.0 / 5.0) + 32.0;
Serial.print(temperatureF);
Serial.print("\xC2\xB0"); // shows degree symbol
Serial.println("F");
delay(1000); // wait a second between readings
}
You can see the result by opening serial monitor:
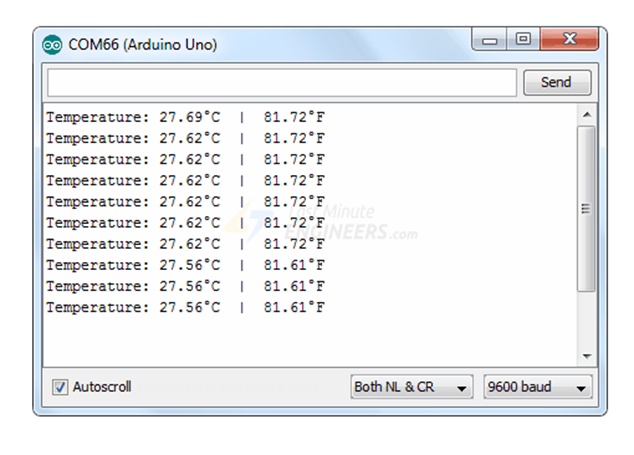
Code Explanation:
First of all we have to define sensor pin connected to A0.

In void setup initialize the serial monitor with hardware.
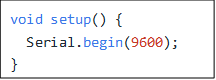
In void loop first read value from sensor using analogRead() function and store the read value in the variable named reading.

Now use formula to convert the read value into voltage and then into temperature that we discussed earlier in the tutorial.
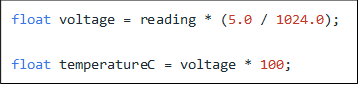
Now showing results on the serial monitor
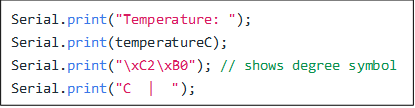
This temperature is in Celsius. We can convert it in Fahrenheit by using simple formula
T (°F) = T (°C) × 9/5 + 32
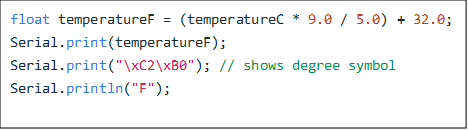
Hello arduinotutors.com webmaster, Good work!