A project involving multiple RFID modules with Arduino can serve many purposes, such as access control systems, inventory management, or attendance tracking. RFID (Radio-Frequency Identification) technology enables wireless communication between an RFID reader and RFID tags. The Arduino microcontroller is an excellent platform to manage multiple RFID modules due to its versatility and ease of programming.
The goal of this project is to create a system where multiple RFID modules can communicate with an Arduino board to perform a variety of tasks such as user authentication, tracking, or monitoring objects with RFID tags. This project is suitable for environments like libraries, offices, warehouses, or schools.
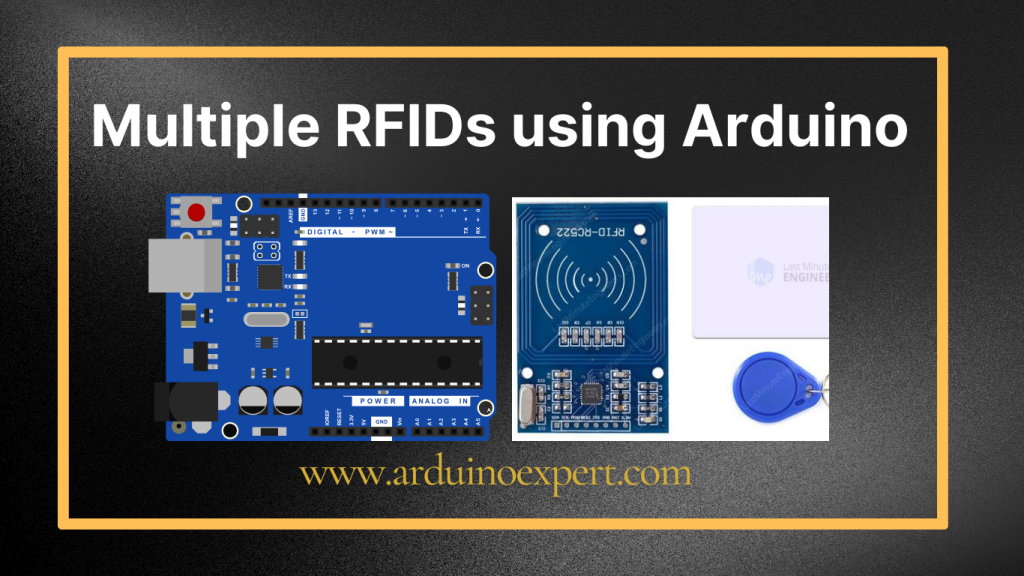
Components used in the Project:
Arduino Board: An Arduino Uno, Mega, or any other compatible board.
RFID Readers: Multiple RFID readers, such as the MFRC522 or RC522, are commonly used with Arduino.
RFID Tags: Passive RFID tags that match the frequency of the RFID readers (typically 13.56 MHz).
Breadboard and Jumper Wires: For making connections between components.
Power Supply: USB power or an external power source for the Arduino.
Relay Modules (Optional): For controlling other devices (e.g., door locks).
Working of Project:
The RFID reader uses electromagnetic fields to automatically identify and track tags attached to objects. When an RFID tag comes within range of an RFID reader, it is energized by the reader’s electromagnetic field and transmits its unique ID back to the reader. The Arduino receives the data from the RFID readers and processes it to perform the desired action, like logging attendance, opening a door, or updating inventory records.
Circuit Diagram:
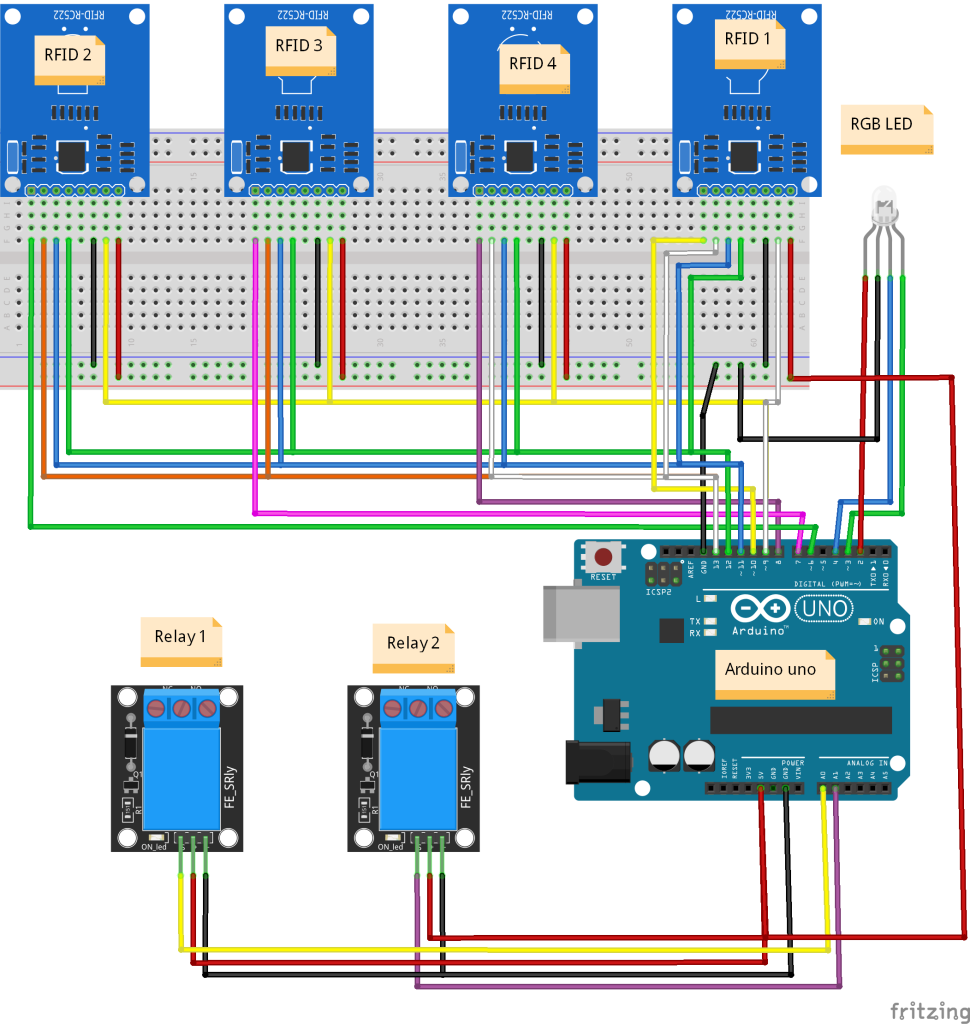
Code:
#include <SPI.h>
#include <MFRC522.h>
#define RST_PIN 9 // Reset pin for all readers
#define SS_PIN1 10 // Slave Select pin for RFID reader 1
#define SS_PIN2 11 // Slave Select pin for RFID reader 2
// Add more SS pins if using more readers
MFRC522 rfid1(SS_PIN1, RST_PIN); // Create an instance for RFID reader 1
MFRC522 rfid2(SS_PIN2, RST_PIN); // Create an instance for RFID reader 2
void setup() {
Serial.begin(9600); // Initialize serial communications
SPI.begin(); // Initialize SPI bus
rfid1.PCD_Init(); // Initialize RFID reader 1
rfid2.PCD_Init(); // Initialize RFID reader 2
Serial.println("Multiple RFID Readers ready.");
}
void loop() {
// Handle RFID reader 1
if (rfid1.PICC_IsNewCardPresent() && rfid1.PICC_ReadCardSerial()) {
Serial.print("RFID Reader 1: ");
printRFID(rfid1);
rfid1.PICC_HaltA();
}
Applications:
Access Control Systems: Secure entry points by using multiple RFID readers at different doors
.Inventory Management: Track items with RFID tags as they move through various stages in a warehouse.
Attendance Monitoring: Automate attendance records for students or employees.
Parking Management: Control and monitor vehicle access in a parking lot.